当前位置:首页> 正文
最新html5游戏开发入门教程知识分享
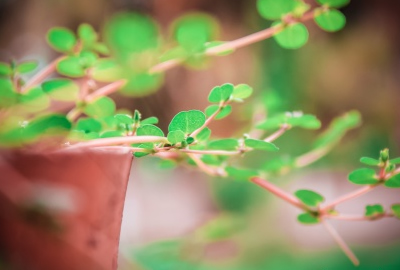
HTML5游戏制作完全指南
简介
你想使用HTML5的Canvas制作一款游戏吗?跟着这个教程,你将立刻上道儿。
阅读该教程需要至少熟悉javascript相关知识。
你可以先玩这款游戏或者直接阅读文章并且下载游戏源码。
创建画布
在画任何东西之前,我们必须创建一个画布。因为这是完全指南,并且我们将用到jQuery.
var CANVAS_WIDTH=480;
var CANVAS_HEIGHT=320;
var canvasElement=$("<canvas width='" + CANVAS_WIDTH +
"' height='" + CANVAS_HEIGHT + "'></canvas>");
var canvas=canvasElement.get(0)。getContext("2d");
canvasElement.appendTo('body');
游戏循环
为了呈现给玩家连贯流畅的游戏动画,我们要频繁地渲染画布来欺骗玩家的眼睛。
var FPS=30;
setInterval(function() {
update();
draw();
}, 1000/FPS);
现在我们先不管update和draw里面的实现,重要的是我们要知道setInterval()会周期性的执行update和draw
Hello world
现在我们已经搭建好了一个循环的架子,我们去修改update和draw方法来写一些文字到屏幕。
function draw() {
canvas.fillStyle="#000"; // Set color to black
canvas.fillText("Sup Bro!", 50, 50);
}
专家提醒: 当你稍微更改了一些代码的时候就执行一下程序,这样可以更快的找到程序出错地方。
静止文字正常的显示出来了。因为我们已经有了循环,所以我们可以很容易地让文字动起来——
var textX=50;
var textY=50;
function update() {
textX +=1;
textY +=1;
}
function draw() {
canvas.fillStyle="#000";
canvas.fillText("Sup Bro!", textX, textY);
}
执行程序。如果你一步一步照着上面做下来,可以看到文字移动。但是上一次的文字却还留在屏幕上,因为我们没有擦除画布。现在我们在draw方法中加入擦除方法。
function draw() {
canvas.clearRect(0, 0, CANVAS_WIDTH, CANVAS_HEIGHT);
canvas.fillStyle="#000";
canvas.fillText("Sup Bro!", textX, textY);
}
现在你可以看到文字在屏幕上移动了,它已经算是一个真正意义上的游戏,只不过是个半成品。
创建player
创建一个包含player所有信息的对象,并且要有draw方法。这里创建了一个简单的对象包含了所有的player信息。
var player={
color: "#00A",
x: 220,
y: 270,
width: 32,
height: 32,
draw: function() {
canvas.fillStyle=this.color;
canvas.fillRect(this.x, this.y, this.width, this.height);
}
};
我们现在用一个纯色的矩形来代表player.当我们把它加入游戏当中的时候,我们需要清除画布并且画上player.
function draw() {
canvas.clearRect(0, 0, CANVAS_WIDTH, CANVAS_HEIGHT);
player.draw();
}
键盘控制
使用jQuery Hotkeys
jQuery Hotkeys plugin在处理键盘行为的时候,可以更加容易的兼容不同的浏览器。让开发者不用因为不同浏览器之间的keyCode andcharCode不同而苦恼,我们这样绑定事件:
$(document)。bind("keydown", "left", function() { … });
移动player
function update() {
if (keydown.left) {
player.x -=2;
}
if (keydown.right) {
player.x +=2;
}
}
是不是感觉移动不够快?那么我们来提高它的移动速度。
function update() {
if (keydown.left) {
player.x -=5;
}
if (keydown.right) {
player.x +=5;
}
player.x=player.x.clamp(0, CANVAS_WIDTH - player.width);
}
我们可以很容易的添加其他元素,比如炮弹:
function update() {
if (keydown.space) {
player.shoot();
}
if (keydown.left) {
player.x -=5;
}
if (keydown.right) {
player.x +=5;
}
player.x=player.x.clamp(0, CANVAS_WIDTH - player.width);
}
player.shoot=function() {
console.log("Pew pew");
// :) Well at least adding the key binding was easy…
};
添加更多游戏元素
炮弹
我们开始真正意义上的添加炮弹,首先,我们需要一个集合来存储它:
var playerBullets=[];
然后,我们需要一个构造器来创建炮弹:
function Bullet(I) {
I.active=true;
I.xVelocity=0;
I.yVelocity=-I.speed;
I.width=3;
I.height=3;
I.color="#000";
I.inBounds=function() {
return I.x >=0 && I.x <=CANVAS_WIDTH &&
I.y >=0 && I.y <=CANVAS_HEIGHT;
};
I.draw=function() {
canvas.fillStyle=this.color;
canvas.fillRect(this.x, this.y, this.width, this.height);
};
I.update=function() {
I.x +=I.xVelocity;
I.y +=I.yVelocity;
I.active=I.active && I.inBounds();
};
return I;
}
当玩家开火,我们需要向集合中添加炮弹:
player.shoot=function() {
var bulletPosition=this.midpoint();
playerBullets.push(Bullet({
speed: 5,
x: bulletPosition.x,
y: bulletPosition.y
}));
};
player.midpoint=function() {
return {
x: this.x + this.width/2,
y: this.y + this.height/2
};
};
修改update和draw方法,实现开火:
function update() {
…
playerBullets.forEach(function(bullet) {
bullet.update();
});
playerBullets=playerBullets.filter(function(bullet) {
return bullet.active;
});
}
function draw() {
…
playerBullets.forEach(function(bullet) {
bullet.draw();
});
}
敌人
enemies=[];
function Enemy(I) {
I=I || {};
I.active=true;
I.age=Math.floor(Math.random() * 128);
I.color="#A2B";
I.x=CANVAS_WIDTH / 4 + Math.random() * CANVAS_WIDTH / 2;
I.y=0;
I.xVelocity=0
I.yVelocity=2;
I.width=32;
I.height=32;
I.inBounds=function() {
return I.x >=0 && I.x <=CANVAS_WIDTH &&
I.y >=0 && I.y <=CANVAS_HEIGHT;
};
I.draw=function() {
canvas.fillStyle=this.color;
canvas.fillRect(this.x, this.y, this.width, this.height);
};
I.update=function() {
I.x +=I.xVelocity;
I.y +=I.yVelocity;
I.xVelocity=3 * Math.sin(I.age * Math.PI / 64);
I.age++;
I.active=I.active && I.inBounds();
};
return I;
};
function update() {
…
enemies.forEach(function(enemy) {
enemy.update();
});
enemies=enemies.filter(function(enemy) {
return enemy.active;
});
if(Math.random() < 0.1) {
enemies.push(Enemy());
}
};
function draw() {
…
enemies.forEach(function(enemy) {
enemy.draw();
});
}
使用图片
player.sprite=Sprite("player");
player.draw=function() {
this.sprite.draw(canvas, this.x, this.y);
};
function Enemy(I) {
…
I.sprite=Sprite("enemy");
I.draw=function() {
this.sprite.draw(canvas, this.x, this.y);
};
…
}
碰撞检测
function collides(a, b) {
return a.x < b.x + b.width &&
a.x + a.width > b.x &&
a.y < b.y + b.height &&
a.y + a.height > b.y;
}
function handleCollisions() {
playerBullets.forEach(function(bullet) {
enemies.forEach(function(enemy) {
if (collides(bullet, enemy)) {
enemy.explode();
bullet.active=false;
}
});
});
enemies.forEach(function(enemy) {
if (collides(enemy, player)) {
enemy.explode();
player.explode();
}
});
}
function update() {
…
handleCollisions();
}
function Enemy(I) {
…
I.explode=function() {
this.active=false;
// Extra Credit: Add an explosion graphic
};
return I;
};
player.explode=function() {
this.active=false;
// Extra Credit: Add an explosion graphic and then end the game
};
加入声音
function Enemy(I) {
…
I.explode=function() {
this.active=false;
// Extra Credit: Add an explosion graphic
};
return I;
};
player.explode=function() {
this.active=false;
// Extra Credit: Add an explosion graphic and then end the game
};
|
展开全文阅读
相关内容
羊城通电脑版|最新版羊城通
羊城通电脑版|最新版羊城通,,1. 最新版羊城通广州的羊城通现在...
提高3A四核羿龙II游戏配置的性能
提高3A四核羿龙II游戏配置的性能,,以节能环保为主题的IT产业,目...
拖拉机类型游戏电脑|拖拉机小游戏单机版
拖拉机类型游戏电脑|拖拉机小游戏单机版,,拖拉机小游戏单机版...
诺基亚最新手机型号|诺基亚手机型号大全诺基亚
诺基亚最新手机型号|诺基亚手机型号大全诺基亚手机,,诺基亚手...
从互联网的背景来看:网民们冒着风险的截图对游戏
从互联网的背景来看:网民们冒着风险的截图对游戏的热爱来自腾...
计算机主板BIOS设置详细-BIOS知识
计算机主板BIOS设置详细-BIOS知识,,什么是电脑BIOS,一般电脑主...
惠普笔记本电脑最新型号|惠普笔记本电脑所有型
惠普笔记本电脑最新型号|惠普笔记本电脑所有型号大全,,1. 惠普...
最新的AMD低端APUa46300盒装处理器上市
最新的AMD低端APUa46300盒装处理器上市,,APU处理器,AMD的APU系...
军用物资9000元z77游戏安装方案
军用物资9000元z77游戏安装方案,,在英特尔Ivy Bridge平台,主板...
vivo最新款超薄手机是哪款
vivo最新款超薄手机是哪款,手机,摄像头,本文目录vivo最新款超...
rpg宝宝快捷键|rpg游戏保存快捷键
rpg宝宝快捷键|rpg游戏保存快捷键,,1. rpg游戏保存快捷键点击...
1394连接是什么1394网络适配器知识
1394连接是什么1394网络适配器知识,,今天有网友在QQ群中问了这...
怎么把电脑显卡清灰分享|显卡清灰教程
怎么把电脑显卡清灰分享|显卡清灰教程,,1. 显卡清灰教程1.首先...
2021电脑系统在哪下载|2021年电脑最新系统
2021电脑系统在哪下载|2021年电脑最新系统,,1. 2021年电脑最新...
499元的全固态高价格的最新r880gmASL板推荐
499元的全固态高价格的最新r880gmASL板推荐,,ASL r880gm 880g...