关于linux:从C程序中执行程序
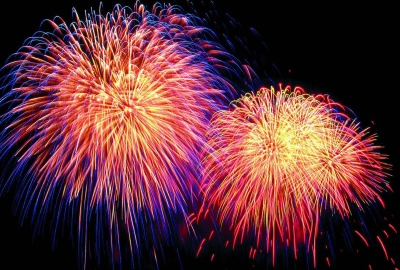
Execute program from within a C program如何在我的 我不确定这是否是标准的C函数。我需要在Linux下可以使用的解决方案。 您要使用 popen是现代unix和类unix操作系统的标准配置,其中Linux是其中之一:-) 类型
在终端上阅读有关它的更多信息。 编辑 我为其他人写了一些示例C代码,展示了如何做到这一点。这是给你的:
子项终止( 对于简单的单向通信,popen()是一个不错的解决方案。但是,它对于双向通信没有用。 国际海事组织(IMO),伊莫赫(Imjorge)(Jorge Ferreira)给出了双向通信的大部分答案(80%?),但省略了一些关键细节。 如果不关闭管道的未使用端,则当其中一个程序终止时,您将不会表现出明智的行为。例如,子级可能正在从其标准输入中进行读取,但是除非在子级中关闭管道的写端,否则它将永远不会得到EOF(读取的零字节),因为它仍然具有打开的管道并且系统认为即使某个管道当前处于挂起状态,正在等待从该管道读取某些内容,有时仍可能会写该管道。 写入过程应考虑是否处理在没有读取过程的管道上写入时给出的SIGPIPE信号。 您必须了解管道容量(取决于平台,可能只有4KB),并设计程序以避免死锁。 您可以使用系统调用,阅读system(3)的联机帮助页。 我认为您可以使用
为此。 |
相关内容
硬盘库存迫切需要通过西方数据,三星已经停止向零
硬盘库存迫切需要通过西方数据,三星已经停止向零售商发送硬盘...
无法读取U盘中的数据
无法读取U盘中的数据,,核心提示:我有一个512MB的U盘,把它插在电...
wps数据拟合图形公式|你好,请问在WPS中拟合线性
wps数据拟合图形公式|你好,请问在WPS中拟合线性方程,请问在WP...
计算机不能打开网页发送更多的数据包,但很少收到
计算机不能打开网页发送更多的数据包,但很少收到(解决方案)。,,问...
wps删除重复数据|WPS表格中,删除重复项,只保留
wps删除重复数据|WPS表格中,删除重复项,只保留一个数据,如何...
如何使用selenium+TestNG做web数据驱动测试
如何使用selenium+TestNG做web数据驱动测试,数据库,数据,本文...
电脑无法启动光驱在响|电脑无法启动光驱在响怎
电脑无法启动光驱在响|电脑无法启动光驱在响怎么办,,电脑无法...
华硕电脑开机进入bios|华硕电脑开机进入bios设
华硕电脑开机进入bios|华硕电脑开机进入bios设置u盘启动,,1. ...
wps表格查找重复的数据|在wps表格中查找重复数
wps表格查找重复的数据|在wps表格中查找重复数据,表格,重复,...
win7怎么看电脑运行时间|win7怎么看软件运行时
win7怎么看电脑运行时间|win7怎么看软件运行时间,,1. win7怎么...
EXCEL数据透视表怎么用?是干什么的
EXCEL数据透视表怎么用?是干什么的,透视,干什么,怎么,excel透视...
最新版Win7系统运行速度非常缓慢怎么解决
最新版Win7系统运行速度非常缓慢怎么解决,最新版,运行速度, ...
电脑进入uefi开机不了系统|电脑uefi启动开不了
电脑进入uefi开机不了系统|电脑uefi启动开不了机,,电脑uefi启...
电脑硬盘启动设置教程|电脑硬盘启动设置方法
电脑硬盘启动设置教程|电脑硬盘启动设置方法,,1. 电脑硬盘启动...
电脑数据的销毁手段|电脑怎么销毁数据
电脑数据的销毁手段|电脑怎么销毁数据,,电脑怎么销毁数据用软...